Unlock the Power of Animated Bar Charts in JavaScript for Your Business Insights
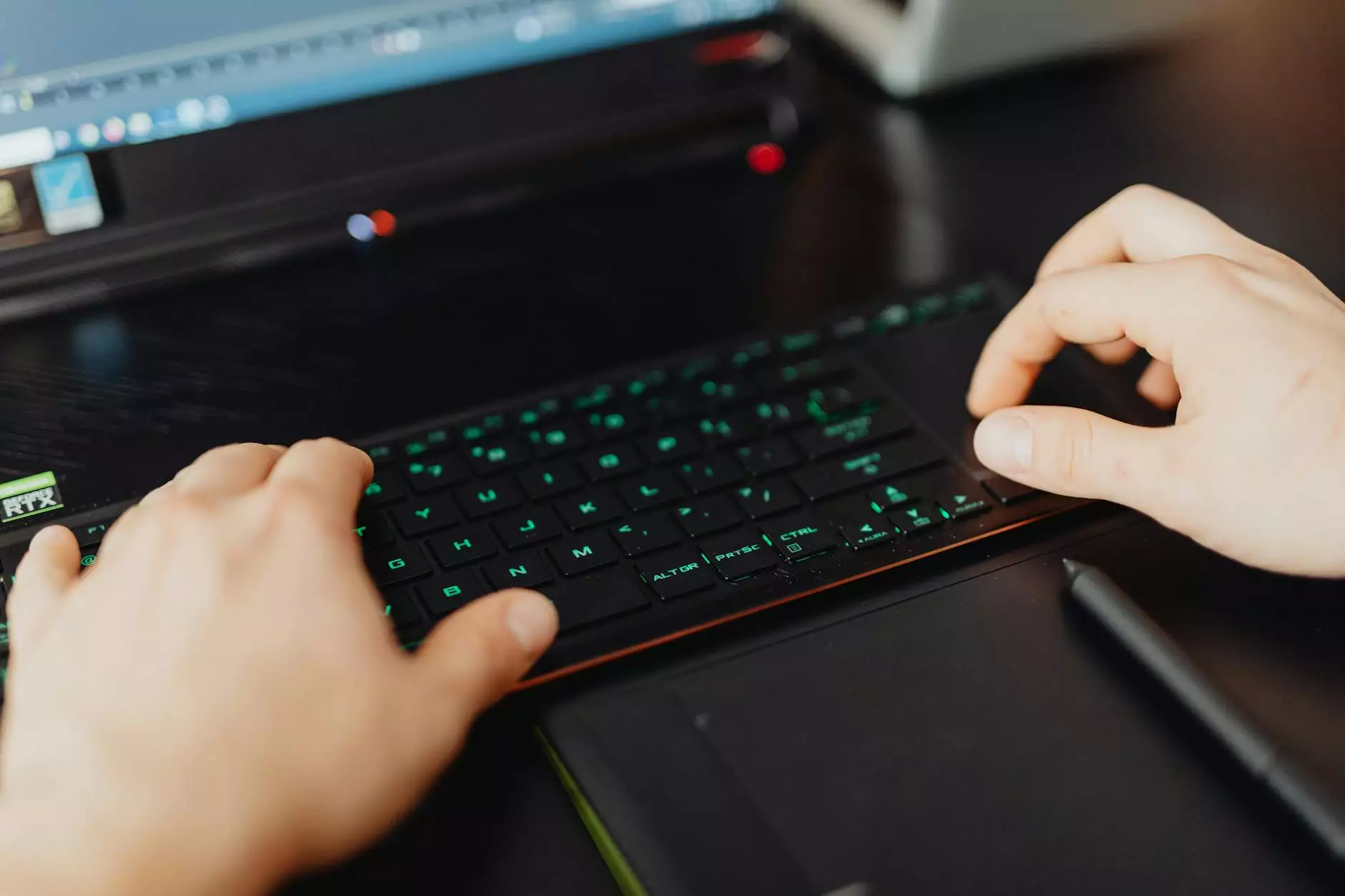
In today's fast-paced digital world, the ability to present data effectively can set your business apart from the competition. One of the most impactful ways to visualize data is through animated bar charts built with JavaScript. This article will explore the importance of data visualization, delve into the mechanics of animated bar charts, and provide you with practical guidance on implementing this powerful tool into your business presentations.
Why Data Visualization Matters
Data visualization is not just a trend; it’s a crucial aspect of modern business operations. The ability to easily interpret complex datasets allows stakeholders to make informed decisions rapidly. Here are some reasons why data visualization, and specifically animated bar charts, are essential:
- Enhanced Understanding: Visual aids simplify the comprehension of data, making it easier to identify trends, patterns, and anomalies.
- Engagement: Animated elements can capture and retain the audience’s attention longer than static visuals, which is particularly important in business presentations.
- Impactful Communication: A well-designed animated bar chart can communicate key insights more effectively than text-heavy reports.
- Aides Memory Retention: Visual data representation aids in memory retention, helping stakeholders to remember critical data points and trends.
The Benefits of Animated Bar Charts
Animated bar charts take traditional data representation a step further. Here’s how they can enhance your business analytics:
- Dynamic Presentation: Animation breathes life into data. It allows the viewer to see changes over time, which static graphs can’t convey adequately.
- Interactive Elements: Often, animated charts come with interactive features that allow users to filter or manipulate data according to their preferences.
- Storytelling with Data: By incorporating animation, you can present a narrative that guides your audience through the data, aligning your message with visual aids.
Getting Started with Animated Bar Charts in JavaScript
To begin using animated bar charts in your projects, you need a basic understanding of JavaScript and a suitable library that facilitates the creation of dynamic graphics. One such popular library is D3.js, which provides extensive functionality for data manipulation and visualization.
Setting Up Your Environment
Before you get into coding, ensure that your development environment is ready. Follow these steps:
- Install a code editor like VS Code or Sublime Text.
- Include the D3.js library in your project. You can either download it or use a CDN link:
Creating Your First Animated Bar Chart
Now that we have the setup ready, let’s go through the steps to create your first animated bar chart.
Step 1: Prepare Your Data
Your data should be in a structured format, such as JSON. For example:
const data = [ { year: "2018", value: 30 }, { year: "2019", value: 80 }, { year: "2020", value: 45 }, { year: "2021", value: 60 }, { year: "2022", value: 100 } ];Step 2: Create the SVG Container
Next, create an SVG container that will hold your bar chart:
const svg = d3.select("body") .append("svg") .attr("width", 500) .attr("height", 300);Step 3: Scale Your Data
To make the chart responsive, scale your data appropriately:
const xScale = d3.scaleBand() .domain(data.map(d => d.year)) .range([0, 500]) .padding(0.1); const yScale = d3.scaleLinear() .domain([0, d3.max(data, d => d.value)]) .range([300, 0]);Step 4: Create Bars
Once your scales are ready, you can create bars with animations:
svg.selectAll(".bar") .data(data) .enter() .append("rect") .attr("class", "bar") .attr("x", d => xScale(d.year)) .attr("y", d => yScale(d.value)) .attr("width", xScale.bandwidth()) .attr("height", d => 300 - yScale(d.value)) .attr("fill", "blue") .transition() .duration(1000) .attr("height", d => 300 - yScale(d.value));Step 5: Adding Axes
Finally, add axes to your chart to make it more informative:
svg.append("g") .attr("class", "x-axis") .attr("transform", "translate(0,300)") .call(d3.axisBottom(xScale)); svg.append("g") .attr("class", "y-axis") .call(d3.axisLeft(yScale));Enhancing Your Chart: Interactivity and More
To make your animated bar chart even more engaging, consider adding interactivity. Here are a few features you can implement:
- Hover Effects: Change the color of bars on hover for immediate visual feedback.
- Tooltips: Display detailed information about each bar when a user hovers over it.
- Responsive Design: Ensure your chart resizes based on the viewport for mobile compatibility.
Best Practices for Implementing Animated Bar Charts
While animated bar charts can be powerful, using them effectively requires knowledge of best practices:
- Keep It Simple: Avoid cluttering your chart with too much data. Focus on key messages you wish to convey.
- Tell a Story: Use animation to guide your audience through the data. Each transition should have a purpose.
- Test for Performance: Ensure that your animation performance is smooth and does not hinder user experience. Optimize your code as necessary.
- Consider Accessibility: Ensure color choices are distinguishable for those with visual impairments, and use text alternatives when necessary.
Conclusion
Implementing animated bar charts using JavaScript can significantly enhance your data presentation capabilities, allowing you to communicate complex information in an engaging and comprehensible manner. By embracing this method of data visualization, you can foster better decision-making and create a lasting impact on your audience.
As you venture into the world of animated charts, remember that practice and continuous learning are key. Explore different datasets, experiment with various design choices, and leverage this tool to elevate your business insights. By doing so, you not only improve the quality of your presentations but also position your business strategically for success in a data-driven marketplace.
animated bar chart js